CS 488/688: Introduction to Computer Graphics
Assignment 3: Animation
Summary
3D computer graphics is not just about generating images of 3D objects. It is equally important to generate an animation of 3D objects. While there are many different ways to define animation of 3D objects, one common approach is to numerically simulate the dynamics of real-world objects according to physics, commonly called physics-based animation. In this assignment, you will implement a simple physics-based animation system.
cs488.h
from A1 and A2 as a starting point. This assignment is mostly dependent on having correct rasterization implemented in A1 or at least a ray-triangle intersection in A2. If you failed all those in A1 and A2 and could not fix any of those to draw something reasonable until now, we can use our reference implementation to grade your code, if necessary. Just being able to rasterize points would be sufficient, though. Make sure to state it in README if it is the case.
Task 0: main.cpp
Comment out A2(argc, argv)
and comment in A3(argc, argv)
to enable a simple setup code in main.cpp
. Here's what you will see.
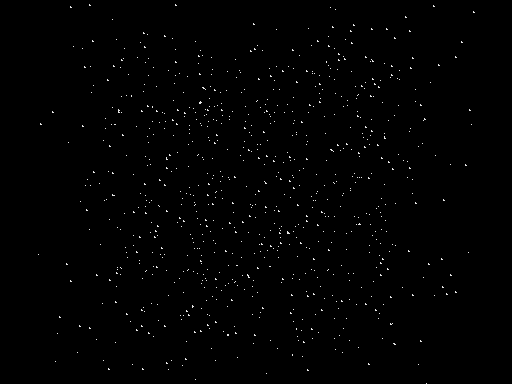
shade
function. Otherwise, your rendering will be extremely slow or broken unless you update the BVH for each frame. You might want to disable illumination computation and return the reflectance to check your results. You can do so by checking globalEnableParticles
and globalRenderType
, for example. Unless your ray tracing code somehow supports efficient rendering of dynamic scenes, you will likely need to stick with the rasterization mode in this assignment.
Task 1: Time integration and gravity
Two classes are relevant for this assignment; Particle
and ParticleSystem
. The Particle
class is about each particle, and the ParticleSystem
is about a collection of particles. The base code already includes some code for rendering particles which renders particles as little triangles that are facing toward the screen. Both positions and velocities of particles are randomly initialized. Fill in the missing part of the Particle::step()
so that the particles now fall under the gravity force defined by float3 globalGravity
with the time step size deltaT
. You will see something like this if it works. You may use any time integration scheme, though we suggest that position-based approaches might be more straightforward for later tasks. Explain what method you use in README.
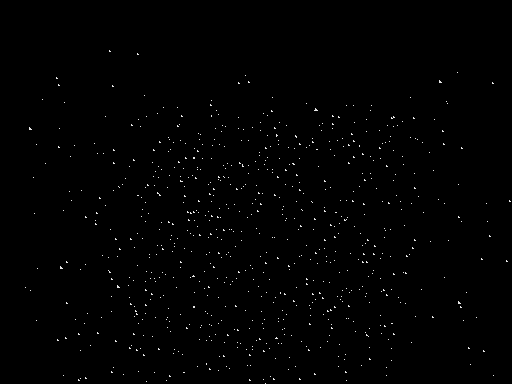
Task 2: Simple collision
Add collision against a box of [-0.5, 0.5] × [-0.5, 0.5] × [-0.5, 0.5] so that particles are contained within this box all the time. Add a proper collision response. Be sure to explain what you did in README.
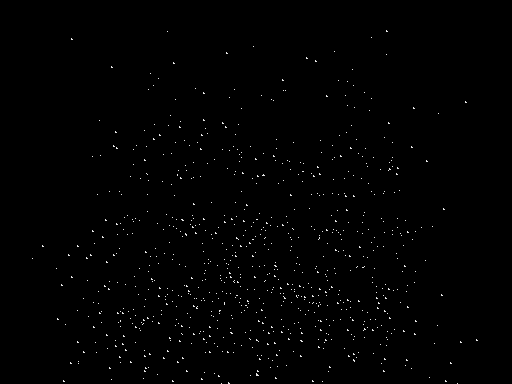
Task 3: Constraints on a spherical surface
Add constraints on the dynamics of particles so that particles are moving only on a spherical surface. Be sure to explain what you did in README. Here is an example. You can turn off the collision in Task 2 if you want.
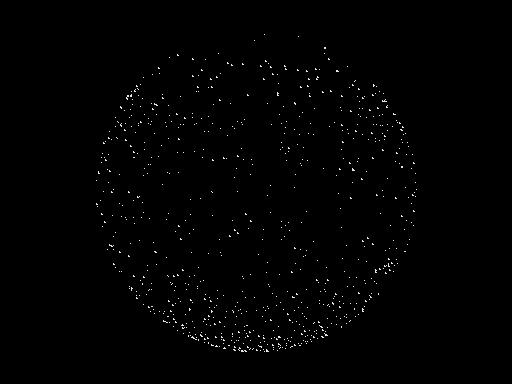
Task 4: Gravitational field
Implement particle-particle interaction based on a gravitational field as explained in the lecture. To access all the particles, you must modify the ParticleSystem
class. Be sure to explain what you did in README. You can turn off the constraint and the collision if you want. Here is an example with the gravity constant times the mass of two particles equals to 2e-3f
.
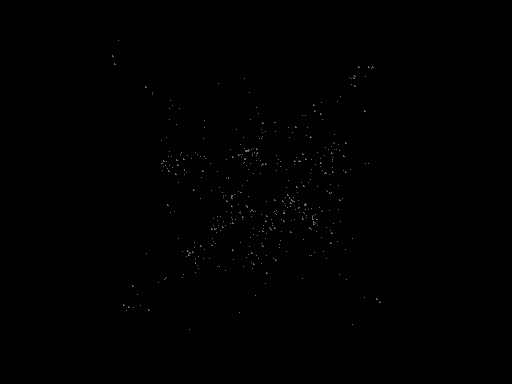
Task 5: Spherical particles
So far, particles are just points. It would be more realistic if each particle were a small sphere. It means that you need to consider the radius of each particle (sphere) into account in your simulation. Add proper collisions so that particles now behave as small spheres. Here is an example together with the constraint in Task 3. You can see that spheres are not perpetrating each other. Turn off the gravitational field in Task 4 to demonstrate your result in Task 5.
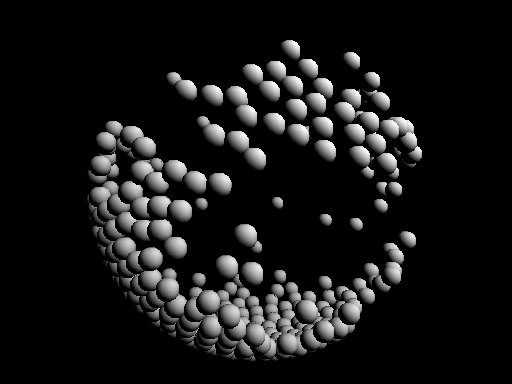
Extras
These are optional extra tasks for those who would like more challenges and potentially gain extra points. Specification of an extra task is intentionally very-high level. Explain what you did in README if you want an extra point. You may or may not receive an extra point depending on our evaluation.
Extra 1: Faster simulation
OK, now you see the pattern. Yes, make your physics simulation as fast as possible. However, for this time, you want to make your system scale well for a larger number of particles because that is usually where your computation increases. How many particles can you handle interactively? Report the performance boost. Consider algorithmic changes such as clustering for multibody dynamics and collision detection. You can also do further optimization except for using other libraries or APIs.
Extra 2: Collision with static triangle meshes
Add collision with static triangle meshes. There should be appropriate collision responses. Triangle meshes do not respond to particle collisions (i.e., they are static). Hint: you can utilize the BVH for the mesh.
What to submit
The submission process for this assignment is the same as the one for Assignment 0. Prepare a ZIP file of your code, omitting unnecessary files (executables, build files, etc.). Upload the ZIP file to LEARN.
You must submit the README
. In this assignment, you must submit videos or animated GIFs to demonstrate what you achieved in each task. Name them as screenshotTask2.gif
etc., to clarify which task you are referring to. If you omit either of the files, you will receive a deduction. See Assignment 0 for instructions about how to prepare these files.
If you do extend the base code, be sure to document your extensions in your README
file. Keep in mind that
you must still satisfy the core objectives listed here. If your changes are so radical that your modified program is
incompatible with the original specification, you must include a "compatibility mode" that makes the interface behave like the requirements here, or consider creating an entirely separate project (but hey, is that making sense?).
If you are using your own .obj file to render images, be sure to include them in your submission. That being said, do not include a too large .obj file.
Other thoughts
Once you complete this assignment, now your system can render dynamic scenes (i.e., particles) with rasterization and render static scenes with either rasterization and ray tracing (or even a hybrid of both!). It is now a good time to finalize your plan for the final project. The due day for the proposal will be within a few days after the deadline of this assignment. There are so many things that you can do on top of it, so think about what you liked throughout the assignments and extend them.
Objectives
Every assignment includes a list of objectives. Your mark in the assignment will be based primarily on the achievement of these objectives, with possible deductions outside the list if necessary.
- Particles fall under the gravity force according to the equation of motion.
- Collision detection and response with the box are working properly.
- Some constraints on the motion of particles are implemented.
- Some form of particle-particle interaction is implemented.
- Spherical particles consider proper collisions among them.